Signature in Java means:An Introduction to Signature in Java
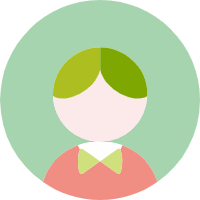
Signature in Java: An Introduction
Signature in Java is a fundamental concept in the programming language, particularly in the context of software development. It is a way to identify the author of a piece of code, ensure its integrity, and protect it from being altered or tampered with. This article provides an overview of the significance of signature in Java, its implementation, and how to use it effectively in your Java projects.
What is a Signature in Java?
A signature in Java is a unique identifier that is associated with a piece of code. It is often used to prevent code from being tampered with or modified without the author's knowledge. A signature can be created using a cryptographic algorithm, such as SHA-256 or SHA-3, which generates a unique hash value for a given piece of code. This hash value is then used as the signature for that code.
Why is a Signature in Java Important?
There are several reasons why a signature in Java is important:
1. Integrity: A signature ensures the integrity of the code by proving that it has not been modified or tampered with since its creation. If the signature changes, it means the code has been modified, and the program may no longer function correctly.
2. Authentication: A signature can be used to authenticate the author of the code. By knowing the author's signature, one can be sure that the code has been written by the stated author.
3. Attribution: A signature can also be used to attribute the work to its rightful author. This can be particularly useful in the context of open-source software development, where developers often contribute code with their signatures.
Implementing a Signature in Java
Implementing a signature in Java involves creating a unique identifier using a cryptographic algorithm and using it to verify the integrity of the code. Here's a simple example of how to create a signature in Java using the SHA-256 algorithm:
```java
import javax.crypto.SHA256Hash;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class SignatureExample {
public static void main(String[] args) {
String input = "This is an example of a signature in Java";
SHA256Hash sha256 = SHA256Hash.getInstance();
byte[] hash = sha256.digest(input.getBytes(StandardCharsets.UTF_8));
String signature = Base64.getEncoder().encodeToString(hash);
System.out.println("Signature: " + signature);
}
}
```
In this example, we have created a signature for the input string "This is an example of a signature in Java" using the SHA-256 algorithm. We then encoded the hash value using Base64 and printed the result as the signature.
Using a Signature in Java
Once you have created a signature for your code, you can use it in various ways, such as:
1. Verifying the Integrity of Code: You can compare the generated signature with the one you created initially to ensure the code has not been tampered with.
2. Authenticating the Author: You can check the author's signature to verify that the code has been written by the stated author.
3. Attributing the Work: You can use the author's signature to attribute the work to its rightful author, particularly in the context of open-source software development.
Signature in Java is a powerful tool that helps ensure the integrity of code, authenticate its author, and attribute the work to its rightful owner. By understanding its significance and implementing it effectively, you can create more secure and reliable software that deserves the trust of your users.