Signature in JavaScript:A Guide to Implementing Signatures in JavaScript Applications
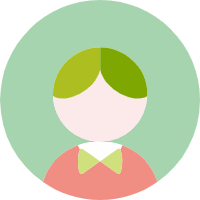
Signatures are a crucial aspect of any programming language, as they provide a way for developers to authenticate and verify the identity of users in their applications. In this article, we will explore the concept of signatures in JavaScript and how to implement them in your JavaScript applications.
1. What are Signatures and Why Are They Important?
Signatures are digital fingerprints that are generated using cryptographic algorithms and used to verify the identity of users in applications. They are often used in conjunction with other authentication methods, such as passwords and two-factor authentication, to provide an additional layer of security.
Implementing signatures in JavaScript applications is important because it adds an additional layer of security to your applications, making it more difficult for attackers to gain access to sensitive data or perform other malicious activities.
2. How to Generate a Signature in JavaScript?
Generating a signature in JavaScript involves using a cryptographic library, such as Web Crypto or crypto-js, to generate a secure random string that can be used as the user's signature. Here's an example using crypto-js:
```javascript
const crypto = require('crypto-js');
const userId = '123456789';
const secretKey = 'your secret key';
const signature = crypto.HMAC(userId, secretKey, 'sha256');
```
In this example, we first require the `crypto-js` package, then generate a HMAC (Harmony Security Message Authentication) using the user's ID and a secret key. The `sha256` algorithm is used as the hashing algorithm, which results in a 64-character string that serves as the user's signature.
3. How to Verify a Signature in JavaScript?
Verifying a signature in JavaScript involves comparing the generated signature with the provided signature to determine if they match. If the signatures match, the user is considered to be the valid owner of the associated account or data. Here's an example using crypto-js:
```javascript
const verified = crypto.hash('sha256', signature) === crypto.hash('sha256', userSignature);
```
In this example, we first use the `hash` function of `crypto-js` to calculate the hash of the provided signature and userSignature. If the hashes match, the `verified` variable will be set to `true`, indicating that the signature has been successfully verified.
4. Best Practices for Implementing Signatures in JavaScript Applications
When implementing signatures in JavaScript applications, it's important to consider the following best practices:
- Choose a strong and unique secret key for each user to generate their signature.
- Store the secret key securely, such as in a encrypted database or in a hard-to-guess environment variable.
- Don't store the user's signature in the clear, as this would make it easy for attackers to generate valid signatures using known information.
- Test your signature verification logic extensively to ensure that it's secure and that it correctly handles different cases, such as invalid signatures or incomplete data.
- Consider using other authentication methods, such as two-factor authentication, in conjunction with signatures to provide an additional layer of security.
Signatures are an essential feature in JavaScript applications, providing an additional layer of security for users and their data. By understanding how to generate and verify signatures in JavaScript, you can create more secure applications that protect your users from potential threats.